반응형
빌더 패턴 이란?
• 복합 객체의 생성 과정과 표현 방법을 분리하여 동일한 생성 절차에서 서로 다른 표현 결과를 만들 수 있게 하는 패턴이다.
• 복합 객체의 생성 과정을 단계별로 분리함으로써 복합 객체의 생성을 일반화 할 수 있다.
• 빌더 패턴의 장점으로 필요한 데이터만 설정 가능, 유연성 확보, 가독성 향상, 불변성 확보가 있다.
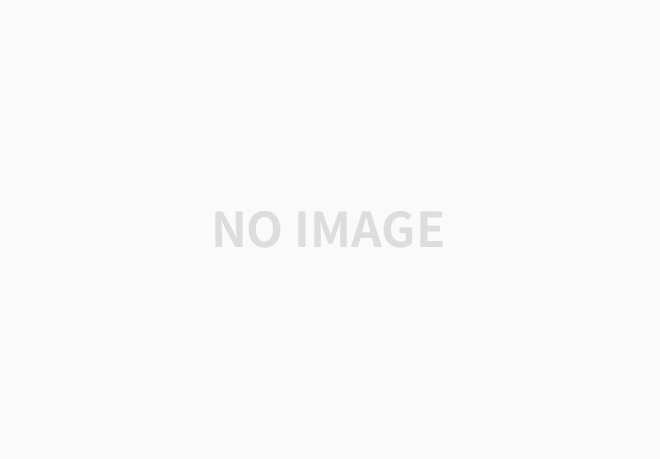
빌더 패턴 구조
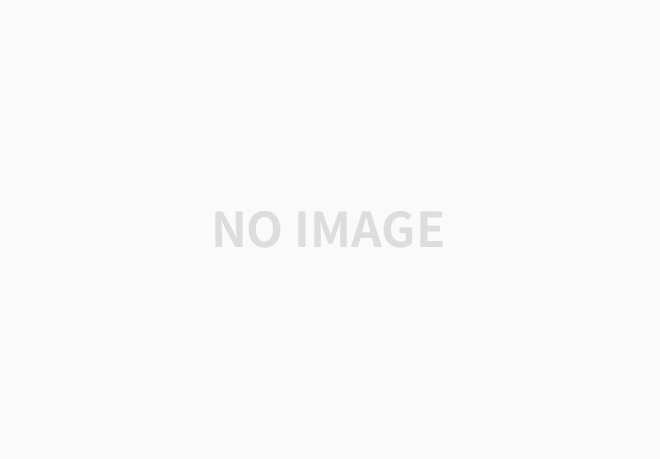
Builder | 객체를 생성하는 추상 인터페이스 |
Concrete Builder | Builder의 구현 클래스. 다른 객체를 생성할 수 있도록 하는 구체적인 클래스 객체를 만들기 위해 부분(부품)을 생성하고 조립한다. |
Director | 객체 생성의 정확한 순서(sequence)를 다루는 부분에 책임이 있다. 이 클래스는 ConcreteBuilder를 인자로 받아서 필요한 동작을 수행한다. |
Product | Builder를 이용해서 Director가 만들어낸 최종 객체 |
사용 예시
빌더 패턴 적용 전
public class Computer {
String cpu;
String hdd;
Integer hddVolume;
String ssd;
Integer ssdVolume;
Boolean bluetoothDongle;
public Computer(String cpu, String hdd, Integer hddVolume, String ssd, Integer ssdVolume, Boolean bluetoothDongle){
this.cpu = cpu;
this.hdd = hdd;
this.hddVolume = hddVolume;
this.ssd = ssd;
this.ssdVolume = ssdVolume;
this.bluetoothDongle = bluetoothDongle;
}
}
// 사용 예시
new Computer("i9-9700k","WD HDD", 1024, null, null, false);
new Computer("i9-9700k", null, null,"T5", 2048, null);
빌더 패턴 적용 후
public class Computer {
private String cpu;
private String hdd;
private Integer hddVolume;
private String ssd;
private Integer ssdVolume;
private String monitor;
private Boolean bluetoothDongle;
private Computer(builder builder){
this.cpu = builder.cpu;
this.hdd = builder.hdd;
this.hddVolume = builder.hddVolume;
this.ssd = builder.ssd;
this.ssdVolume = builder.ssdVolume;
this.monitor = builder.monitor;
this.bluetoothDongle = builder.bluetoothDongle;
}
public static class builder {
// Essential value
private final String cpu;
private final String hdd;
private final Integer hddVolume;
// Selective value
private String ssd;
private Integer ssdVolume;
private String monitor;
private Boolean bluetoothDongle;
public builder(String cpu,String hdd,Integer hddVolume){
this.cpu = cpu;
this.hdd = hdd;
this.hddVolume = hddVolume;
}
public builder ssd(String ssd, Integer ssdVolume){
this.ssd = ssd;
this.ssdVolume = ssdVolume;
return this;
}
…
public Computer build(){
return new Computer(this);
}
}
}
public class TestComputer {
public static void main(String[] args) {
Computer computer = new Computer.builder("i7", "HDD", 1024)
.ssd("T7", 1024)
.monitor("27 inch")
.bluetoothDongle(true)
.build();
}
}
반응형
'객체 지향 프로그래밍 > 디자인 패턴' 카테고리의 다른 글
[구조 패턴] 퍼사드 패턴 (Façade Pattern) 이란? (0) | 2022.05.30 |
---|---|
[구조 패턴] 데코레이터 패턴 (Decorator Pattern) 이란? (0) | 2022.05.26 |
[생성 패턴] 팩토리 메서드 패턴 (Factory Method Pattern) 이란? (0) | 2022.05.26 |
[생성 패턴] 싱글톤 패턴 (Singleton Pattern) 이란? (0) | 2022.05.26 |
댓글